React has become a go-to library for building interactive and efficient user interfaces. Whether you’re a beginner in web development or a seasoned programmer, understanding how to run a React app is a vital skill. This guide walks you through the entire process—from setting up your environment to troubleshooting errors.
React’s flexibility allows you to create dynamic, fast, and responsive applications for web and mobile. But before you can unleash its power, you need to know the steps to run a React app seamlessly. Here, we’ll break down the process into digestible parts so you can confidently run React projects.
Why Learning How to Run a React App Matters
React is one of the most popular JavaScript libraries for building UI components. Knowing how to run a React app ensures that you can effectively test, debug, and deploy applications. Here are three reasons why this knowledge is essential:
- Efficient Development: React’s live reload feature allows developers to see changes instantly.
- Wide Applicability: React is used in various domains, from websites to mobile apps.
- Career Growth: Companies like Facebook, Netflix, and Airbnb rely on React for their user interfaces.
Step-by-Step Guide on How to Run a React App
Setting Up Your Development Environment
Before running a React app, ensure your environment is ready:
- Install Node.js: Download Node.js from the official website.
- Install npm or yarn: npm is included with Node.js, but you can install Yarn for package management.
- Install a Code Editor: Visual Studio Code (VS Code) is a popular choice for React developers.
Creating a New React App
To create a new React app, follow these steps:
- Open your terminal or command prompt.
- Run the command:
npx create-react-app my-app
. - Navigate into your project directory:
cd my-app
. - Use
npm start
to run the app on a local server.
These steps ensure you have the necessary boilerplate to start development.
How to Run a React App in VS Code
Using VS Code makes running a React app intuitive:
- Open the project folder in VS Code.
- Install the ES7+ React/Redux/React-Native snippets extension for React-specific shortcuts.
- Use the integrated terminal to run the app:
npm start
. - The app will open in your default web browser at
http://localhost:3000
.
Exploring the React Folder Structure
Understanding the folder structure is crucial for running and modifying a React app:
- src Folder: Contains the core application files.
- public Folder: Hosts static files like
index.html
. - package.json: Lists project dependencies and scripts.
Knowing these folders will help you organize your code effectively.
Debugging Common Errors
While running a React app, you might encounter issues. Here’s how to resolve them:
- Syntax Errors: Use the terminal logs to locate the problem.
- Module Not Found: Run
npm install
to ensure all dependencies are installed. - Port Conflicts: Use
npm start --port 3001
to change the default port.
Running a React App on Different Platforms
React apps can run on various platforms:
- Local Development Server: Use
npm start
for live testing. - Hosting Services: Deploy your app on services like Netlify or Vercel.
- Mobile: Use React Native for building cross-platform mobile apps.
How to Run a React Project from GitHub
To run a project from GitHub:
- Clone the repository using
git clone <repository-url>
. - Navigate to the project folder:
cd project-folder
. - Install dependencies with
npm install
. - Run the app using
npm start
.
Optimizing Performance
Optimizing a React app ensures a smooth user experience:
- Code Splitting: Use dynamic imports to load components as needed.
- Lazy Loading: Optimize images and large components for faster load times.
- Minification: Use tools like Webpack to compress files.
Using Advanced Features in React
React offers advanced features for improved functionality:
- React Hooks: Simplify state management and lifecycle events.
- Context API: Share data between components without props drilling.
- Custom Components: Create reusable components for consistent design.
Preparing for Deployment
Before deploying, ensure your app is production-ready:
- Run
npm run build
to create an optimized production build. - Use hosting services like AWS, Firebase, or Heroku.
- Test the app thoroughly to ensure functionality.
Conclusion: How to Run a React App
Understanding how to run a React app is a fundamental skill for any developer. Whether you’re setting up a new project, troubleshooting errors, or deploying your app, following these steps will help you succeed.
React’s versatility and power make it a valuable tool in the modern developer’s toolkit. With this guide, you’ll be well-equipped to create, run, and optimize React applications. So, roll up your sleeves and start coding your way to success!
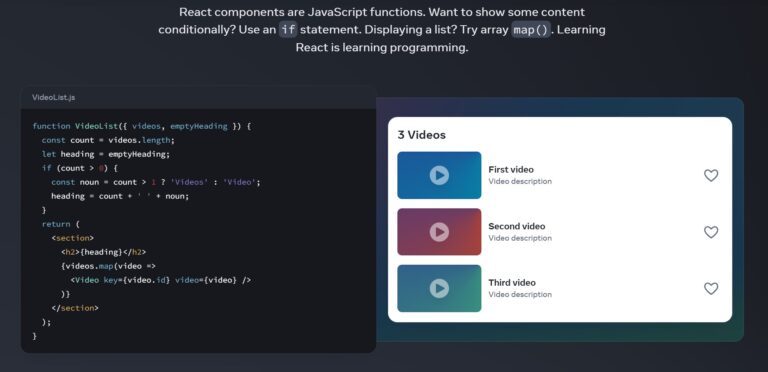